Overview
In order to perform a basic type of catalog data export, you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
As soon as you do authentication and receive a valid token, it needs to be passed on the call to the server.
Endpoint
Example for such endpoint for TT server is https://tt.api.sellercloud.com/rest/api/Catalog/Exports/Basic
Request
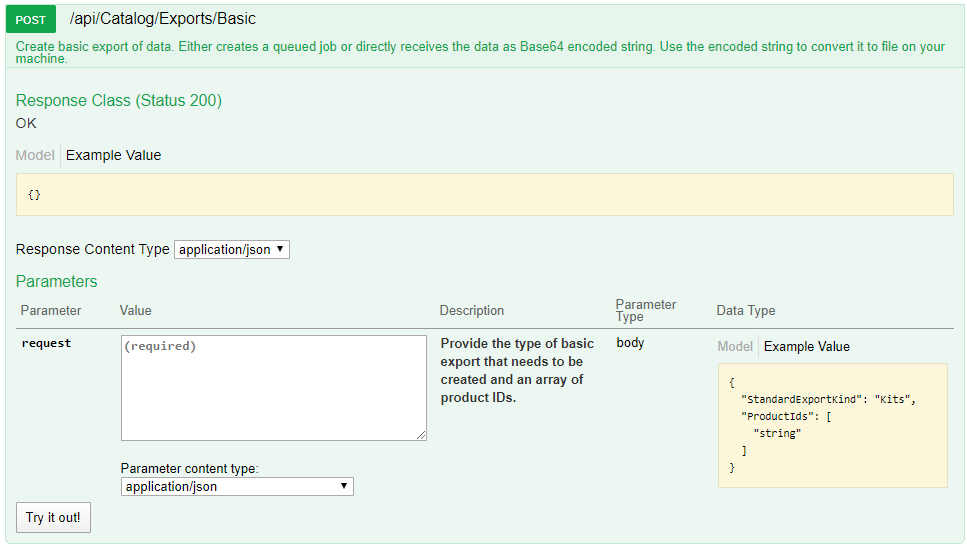
- Method Type: HttpPost
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
Request Model
Field Name | Type | Description |
StandardExportKind | integer | Type of the basic export to be performed. Mandatory.
Possible export types can be found at the end of the article. |
ProductIds | Array of strings | Pass the product SKUs to export data for as an array of strings. Mandatory. |
Response
- If user is authenticated then response will be Status Code 200 => OK.
Some of the exports will generate a queued job and the response model will contain the queued job link.
For other types of basic exports the response contains the name of the generated file and its contents in the form of a Base64 encoded string. From the encoded string you can generate the file which contains exported product data. - If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Exported Types
public enum StandardExportProductInfoKind { Kits = 0, Shadows = 1, Aliases = 2, Variations = 3, Rebates = 4, GeneralExportInExcel = 5, GeneralExportInPdf = 6, RelatedProducts = 7, RelatedKits = 8, ProductGroups = 9, ListedOnChannel = 10, ProductImages = 11, UpcForChannel = 12, VendorSkus = 13 }
Demo in C#
string token = "test_token"; string url = $"http://cwa.api.sellercloud.com//api/Catalog/Exports/Basic"; var content = new BasicExportRequest() { ProductIds = new string[] { "test" }, StandardExportKind = 5 }; using (var client = new HttpClient()) using (var request = new HttpRequestMessage(HttpMethod.Post, url)) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); var json = JsonConvert.SerializeObject(content); using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json")) { request.Content = stringContent; HttpResponseMessage responseMessage = await client.SendAsync(request); var jobLink = await responseMessage.Content.ReadAsStringAsync(); } }