Overview
In order to do product export via plugin, you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
As soon as you do authentication and receive a valid token, it needs to be passed on the call to the server.
Plugins
Getting available plugins can be done from here:
https://tt.api.sellercloud.com/rest/api/Catalog/Exports/ViaPlugin/Metadata
Current example is for TT server. For your server it will be:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Exports/ViaPlugin/Metadata
Plugin will be used for doing products export.
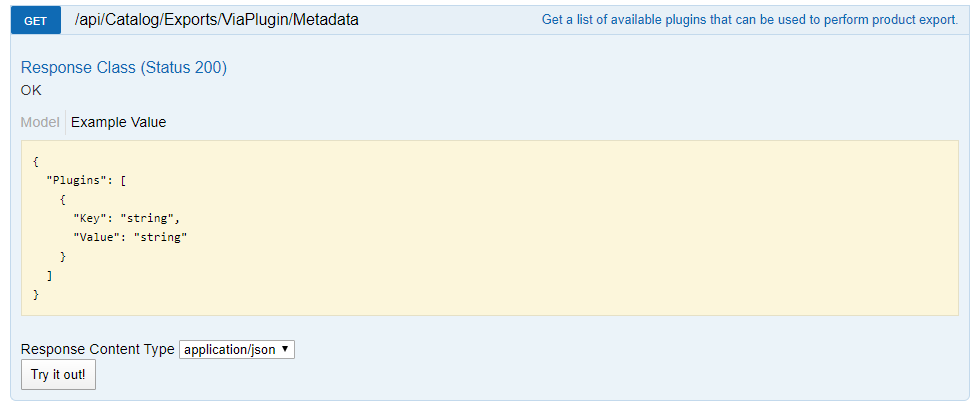
Endpoint for exporting via Plugin
Example for such endpoint for TT server is https://tt.api.sellercloud.com/rest/api/Catalog/ViaPlugin
For your server:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/ViaPlugin
Request
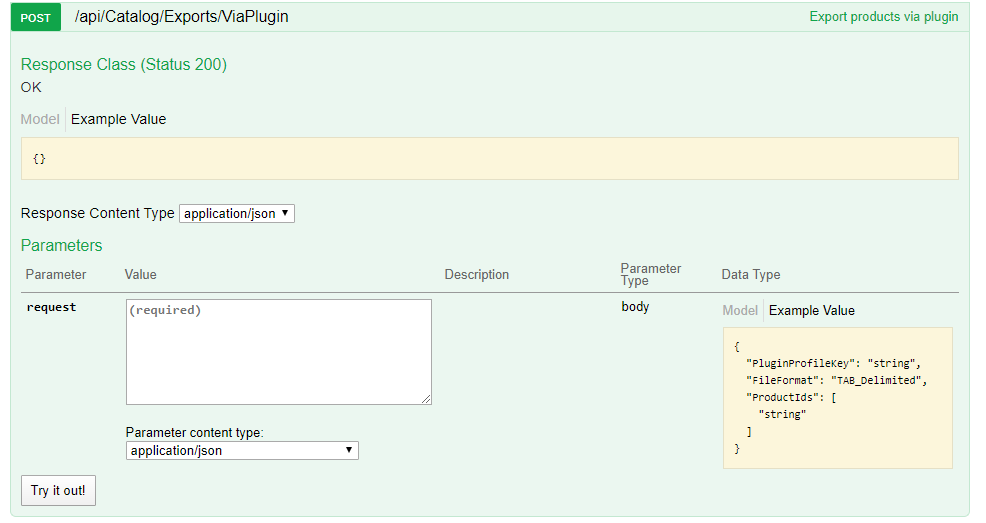
- Method Type: HttpPost
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
Request Model
Field Name | Type | Description |
PluginProfileKey | string | Plugin key received from above endpoint. |
FileFormat | string | File format type of exported data.
TAB_Delimited = 0 CSV = 1 Excel = 2 |
ProductIds | Array of strings | List of product SKUs. |
Response
- If user is authenticated then response will be Status Code 200 => OK and a queued job link.
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Demo in c#
private static string token = "test token"; static async Task GetFirstPlugin() { string url = $"http://cwa.api.sellercloud.com/api/Catalog/Exports/ViaPlugin/Metadata"; using (HttpClient client = new HttpClient()) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); HttpResponseMessage responseMessage = await client.GetAsync(url); var content = responseMessage.Content .ReadAsStringAsync() .Result; var profiles = JsonConvert.DeserializeObject(content); return profiles.Plugins .First() .Key; } } static async Task Export(string plugin) { string url = $"http://cwa.api.sellercloud.com/api/Catalog/Exports/ViaPlugin"; var content = new ExportRequest() { ProductIds = new string[] { "test" }, PluginProfileKey = plugin, FileFormat = FileFormat.Excel }; using (var client = new HttpClient()) using (var request = new HttpRequestMessage(HttpMethod.Post, url)) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); var json = JsonConvert.SerializeObject(content); using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json")) { request.Content = stringContent; HttpResponseMessage responseMessage = await client.SendAsync(request); var jobLink = await responseMessage.Content.ReadAsStringAsync(); } } } static async Task Main(string[] args) { var plugin = await GetFirstPlugin(); var jobLink = await Export(plugin); }