Overview
This endpoint is for importing catalog-related data into Sellercloud via the Rest API. Our REST API provides a host of options that are designed to simplify your data import process.
Our REST API allows you to do many of the same kinds of imports as the Import Catalog Info feature in the new Sellercloud UI. You can learn more about that feature in this helpsite article.
Supported Import Types
The REST API support 6 different import types related to Catalog data:
- Bulk Update Products, which can be used for creating products and updating existing products in your Sellercloud account. See this article for more information about this feature in the Sellercloud UI. Note: This is not intended for updating inventory in Sellercloud.
- Variations, which can be used for creating new variations (matrices) in bulk, or adding products to an existing variation (matrix) in bulk. See this article for more information about this feature in the Sellercloud UI.
- Shadows. See this article for more information about this feature in the Sellercloud UI.
- Kits. See this article for more information about this feature in the Sellercloud UI.
- Product Images. See this article for more information about this feature in the Sellercloud UI.
- Variation Images. See this article for more information about this feature in the Sellercloud UI.
In order to perform any one of the above import types via API, you should first download a dummy template and populate it with the information that will be imported. Downloading a template should be done in the SellerCloud UI. See the linked articles above for help with producing a template in the SellerCloud UI.
1) Bulk Update Products
In order to do bulk update, you have to first download a dummy template from SellerCloud UI in one of the formats:
- TAB Delimited
- CSV
- Excel
You can choose the file format and the columns that you want to use. In the following video we show how you can define your own custom template. Later it can be used for importing different kind of product info in bulk via the REST API
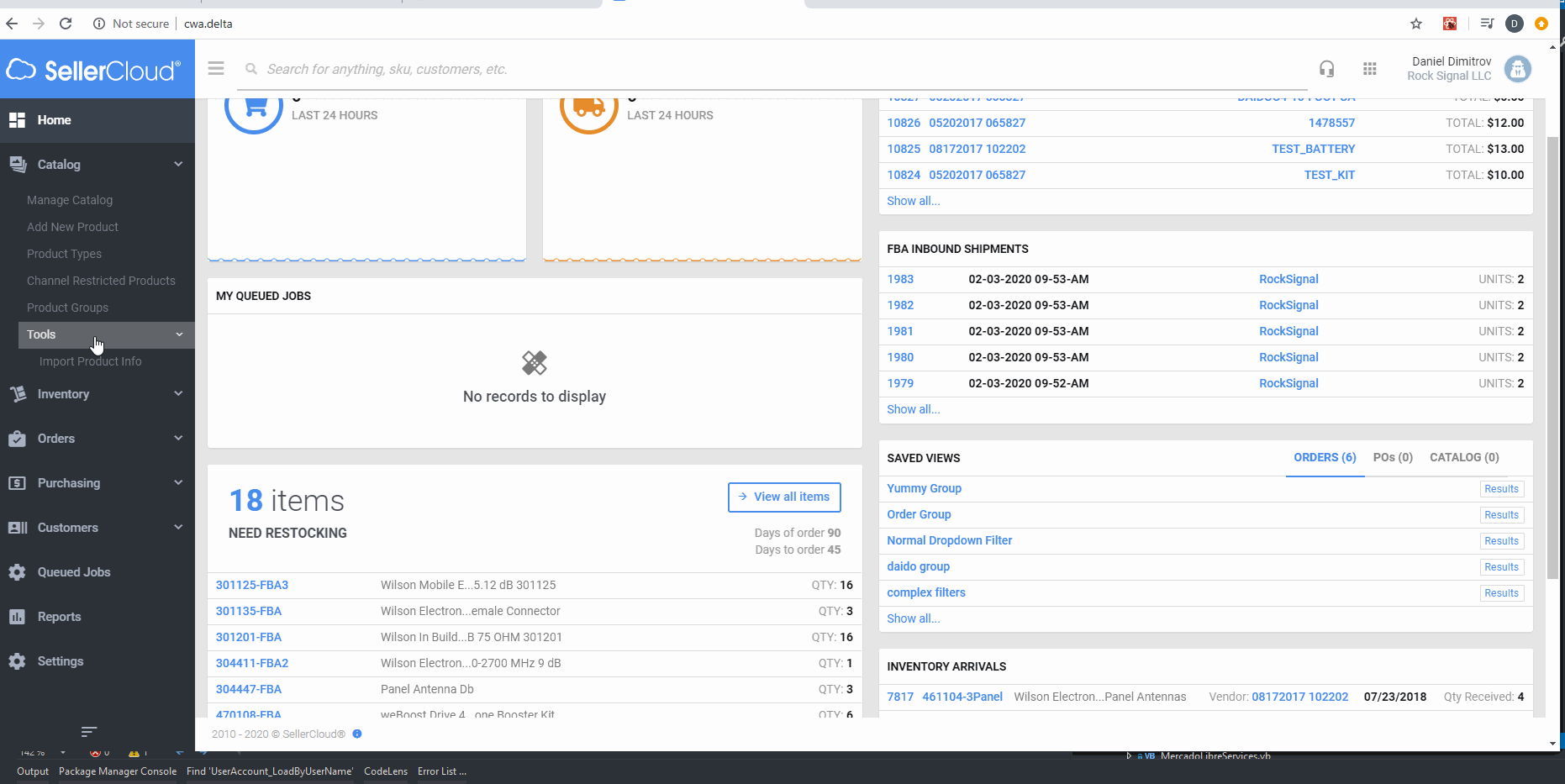
After the template file is downloaded and populated with catalog info (either manually or via code), you can proceed with importing that file via the REST API.
The endpoint for bulk update for the TT server is:
https://tt.api.sellercloud.com/rest/api/Catalog/Imports/Custom
The endpoint for another server will be:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Custom
More information about the endpoint can be found in Swagger as well:
- Method Type: HttpPost
- Authorization: Use Bearer Token + token received from token authentication
- Header Info: Content-Type: application/json
- Body with information for the updated products in bulk
Parameter | Data Type | Description | Is Required |
Format | int | Format of the file that holds information for updated products. Mandatory.
Tab Delimited = 0 CSV = 1 Excel = 2 |
Yes |
FileContents | string | Content of the file in binary format and Base 64 encoded. Mandatory. | Yes |
Metadata | object | Hold information about how to proceed with the new products, schedule task information etc. Mandatory
Format: “Metadata“: { “ScheduleDate“: “”, “CreateProductIfDoesntExist“: true, “CompanyIdForNewProduct“: 1, “UpdateFromCompanyId“: 0 }, ScheduleDate: At what time task to be scheduled. Not Mandatory. CreateProductIfDoesntExist: Boolean. Indicates if new products should be added in SellerCloud if they don’t exist. Not mandatory. If not set, non-existing products will not be created in SellerCloud. CompanyIdForNewProduct: Integer. ID of company. New products that are created from the file import will be linked to that company. DoNotUpdateExistingProducts: Boolean. Indicates if found products to be updated. Not mandatory. If not set, found products will be updated. UpdateFromCompanyId: Integer. ID of company. Sets which company’s products should be updated (for already-existing products). If you input “0”, all companies will be checked. |
Yes |
FileExtension | string | The file extension of the encoded file. Some possible values are: .xls .xlsx .txt .csvTypically optional, but required when file extension is .xlsx |
No |
Important!
Keep in mind that if you are going to create new products via the bulk update, some metadata is required and you need to provide it in the template:
- If client setting “Require SiteCost and Buyer/Purchaser while creating product” is enabled, then site cost and purchaser are required for the new product.
- If client setting “Require Manufacturer when creating Product.” is enabled, then manufacturer must be provided for the new product.
Response
- If user is authenticated and provides a valid information in the request, then response will be Status Code 200 => OK and a link to the newly generated queued job.
- If user is not authenticated, then response will be Status Code 401 => Unauthorized
- On server error => Status Code 500 => Internal Server Error
Example Response
{ "QueuedJobLink": "Your bulk update has been queued. Job # 28163. Click here To monitor Schedule" "ID": 283163 }
Example Demo in C#
public enum FileFormatType { TAB_Delimited = 0, CSV = 1, Excel = 2 }
public class BulkUpdateMetadata { public DateTime? ScheduleDate { get; set; }
public bool CreateProductIfDoesntExist { get; set; }
public int CompanyIdForNewProduct { get; set; }
public int UpdateFromCompanyId { get; set; }
public bool DoNotUpdateProducts { get; set; }
}
public class Request { public BulkUpdateMetadata Metadata { get; set; }
public string FileContents { get; set; }
public FileFormatType Format { get; set; }
}
string url = $"http://cwa.api.sellercloud.com/api/Catalog/Imports/Custom"; string token = "test_token"; int companyID = 1;
var content = new BulkUpdateProductRequest()
{
FileContents = Convert.ToBase64String(File.ReadAllBytes($@”C:DataTestRunsBulk.xls”)),
Format = FileFormatType.Excel,
Metadata = new BulkUpdateMetadata()
{
CompanyIdForNewProduct = companyID
}
};
using (var client = new HttpClient())
using (var request = new HttpRequestMessage(HttpMethod.Post, url))
{
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue(“Bearer”, token);
var json = JsonConvert.SerializeObject(content);
using (var stringContent = new StringContent(json, Encoding.UTF8, “application/json”))
{
request.Content = stringContent;
await client.SendAsync(request);
}
}
2) Variations Import
Downloading a template for importing variations can happen from Sellercloud UI and from REST API.
Endpoint For Downloading Variations Template
Example for such endpoint for TT server is https://tt.api.sellercloud.com/rest/api/Catalog/Imports/Variations/Template?fileFormat=2
For your server endpoint will be:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Variations/Template?fileFormat=2
Request for Downloading Variations Template
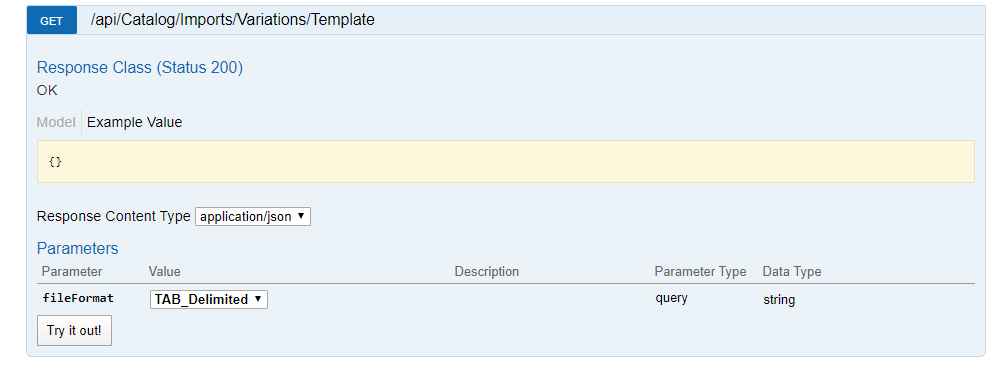
enum FileFormatType { TAB_Delimited = 0 CSV = 1 Excel = 2 }
Response for downloading variations template
- If user is authenticated then response will be Status Code 200 => OK
Content of the response will be Base-64 encoded array of bytes, that represent the file content. Client needs to decode and use the bytes array.
Name of the file can be found in the response content header Content-Disposition.
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Demo in c# for downloading variations template
static async Task DownloadingVariationTemplate() { string token = "test_token"; string url = $"localhost:8080/api/Catalog/Imports/Variations/Template?fileFormat=2";
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization =
new AuthenticationHeaderValue(“Bearer”, token);
HttpResponseMessage responseMessage = await client.GetAsync(url);
var content = responseMessage.Content
.ReadAsStringAsync()
.Result;
var contentInBytes = Convert.FromBase64String(content);
System.IO.File.WriteAllBytes($@”C:DataTestRuns{responseMessage.Content.Headers.ContentDisposition.FileName}”, contentInBytes);
}
}
Request for importing variations
Endpoint for importing variations is:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Variations
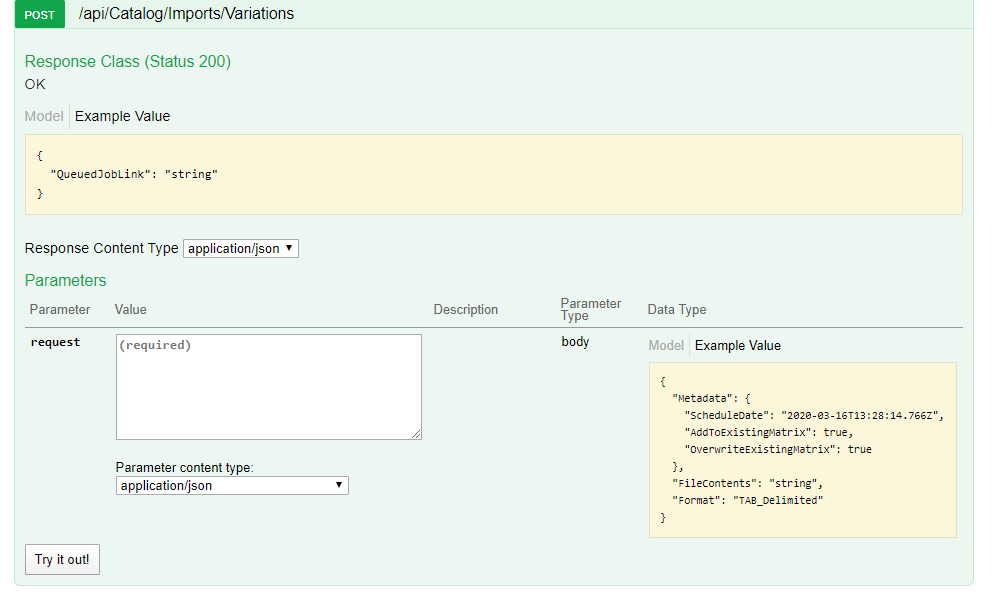
3) Shadows Import
Downloading a template that will be used for importing shadows can be done from SellerCloud UI and from REST API.
Endpoint for downloading shadows template
Example for such endpoint for TT server is https://tt.api.sellercloud.com/rest/api/Catalog/Imports/Shadows/Template?fileFormat=2
For your server endpoint will be:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Shadows/Template?fileFormat=2
Request for downloading shadows template
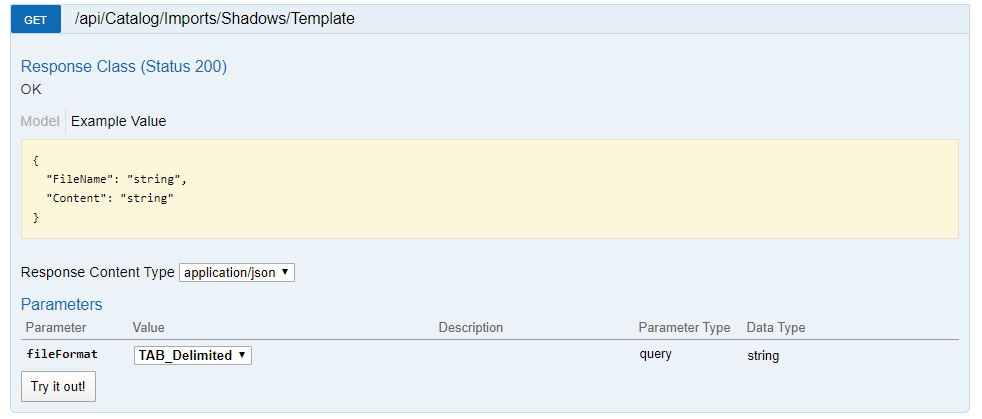
Response from downloading shadows template
- If user is authenticated then response will be Status Code 200 => OK
Content of the response will be Base-64 encoded array of bytes, that represent the file content. Client needs to decode and use the bytes array.
Name of the file can be found in the response content header Content-Disposition.
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Demo in c# for downloading shadows template
static async Task DownloadingShadowsTemplate() { string token = "test_token"; string url = $"localhost:8080/api/Catalog/Imports/Shadows/Template?fileFormat=2";
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization =
new AuthenticationHeaderValue(“Bearer”, token);
HttpResponseMessage responseMessage = await client.GetAsync(url);
var content = responseMessage.Content
.ReadAsStringAsync()
.Result;
var contentInBytes = Convert.FromBase64String(content);
System.IO.File.WriteAllBytes($@”C:DataTestRuns{responseMessage.Content.Headers.ContentDisposition.FileName}”, contentInBytes);
}
}
Request for importing shadows
Endpoint for importing shadows is:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Shadows
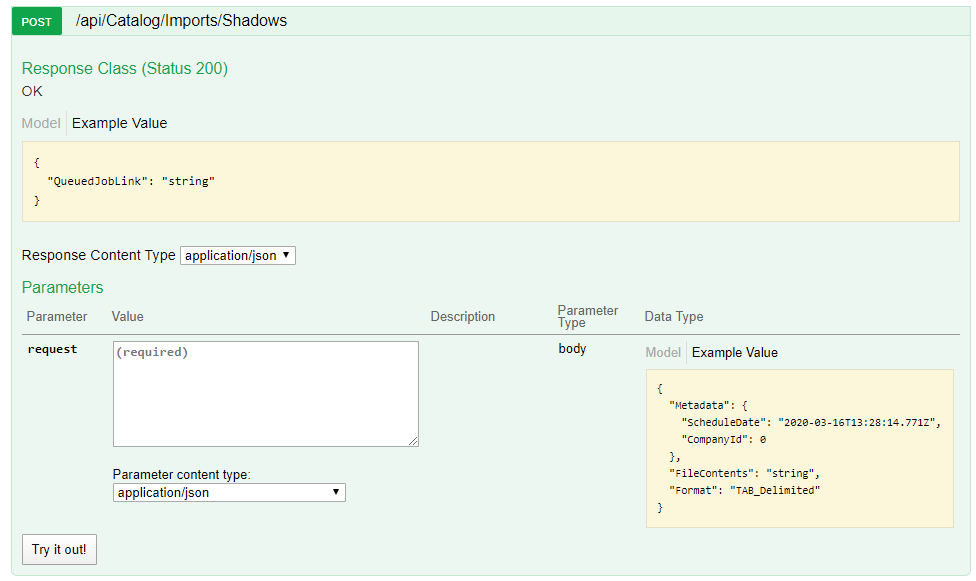
4) Kits Import
Downloading a template that will be used for importing kits can be done from SellerCloud UI and from REST API.
Endpoint for downloading kit template
Example for such endpoint for TT server is https://tt.api.sellercloud.com/rest/api/Catalog/Imports/Kits/Template?fileFormat=2
For your server endpoint will be:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Kits/Template?fileFormat=2
Request for downloading kit template
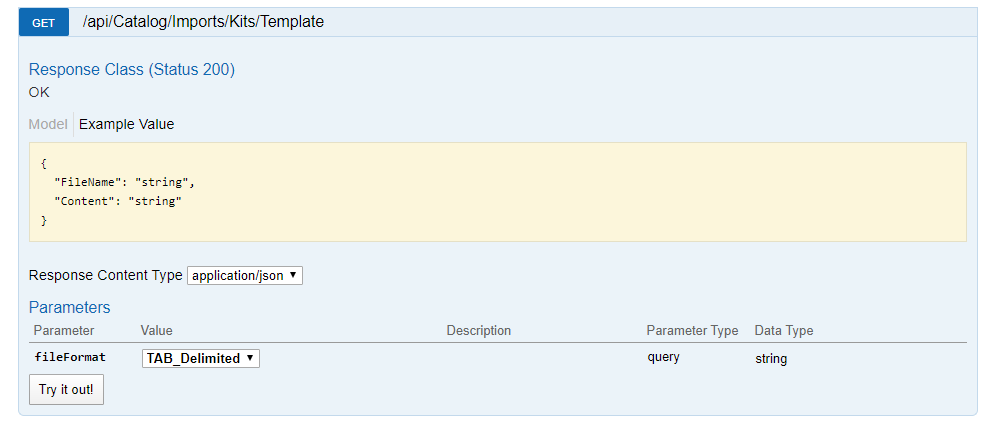
Response when downloading kits template
- If user is authenticated then response will be Status Code 200 => OK
Content of the response will be Base-64 encoded array of bytes, that represent the file content. Client needs to decode and use the bytes array.
Name of the file can be found in the response content header Content-Disposition.
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Request for importing kits
Endpoint for importing kits is:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Kits
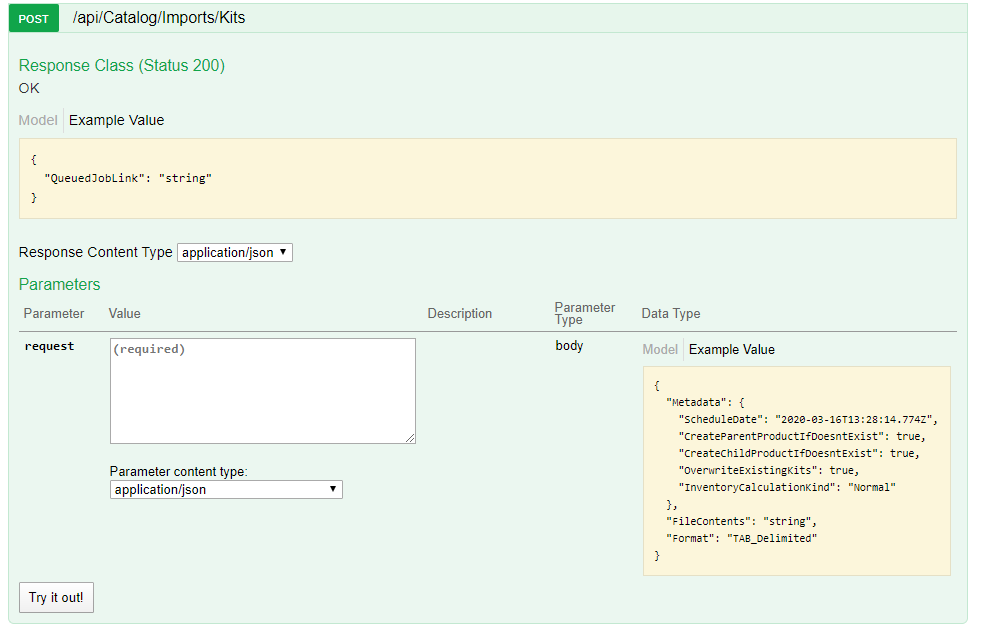
5) Product Images Import
Downloading a template that will be used for importing product images can be done from SellerCloud UI and from REST API.
Endpoint for downloading product images template
Endpoint for downloading product images template is:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Images/Template
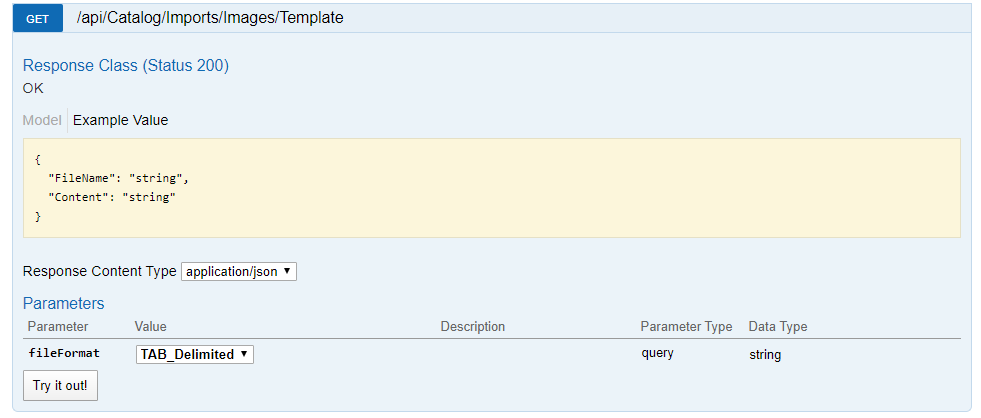
Response when downloading product images template
- If user is authenticated then response will be Status Code 200 => OK
Content of the response will be Base-64 encoded array of bytes, that represent the file content. Client needs to decode and use the bytes array.
Name of the file can be found in the response content header Content-Disposition.
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Demo in c# for downloading variations images template
static async Task DownloadingShadowsTemplate() { string token = "test_token"; string url = $"localhost:8080/api/Catalog/Imports/Images/Template?fileFormat=2";
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization =
new AuthenticationHeaderValue(“Bearer”, token);
HttpResponseMessage responseMessage = await client.GetAsync(url);
var content = responseMessage.Content
.ReadAsStringAsync()
.Result;
var contentInBytes = Convert.FromBase64String(content);
System.IO.File.WriteAllBytes($@”C:DataTestRuns{responseMessage.Content.Headers.ContentDisposition.FileName}”, contentInBytes);
}
}
6) Variations Images Import
Downloading a template that will be used for importing variations images can be done from SellerCloud UI and from REST API.
Endpoint for downloading variations images template
Endpoint for downloading variations images template is:
https://{server_id}.api.sellercloud.com/rest/api/Catalog/Imports/Variations/Images/Template
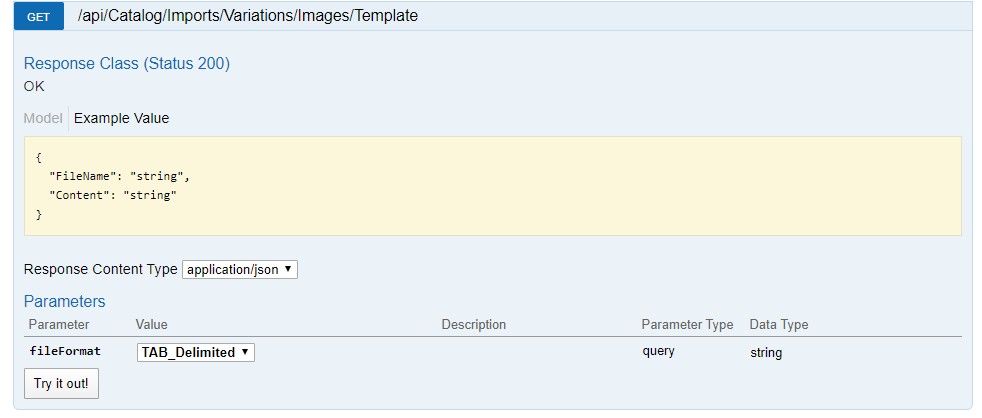
Response when downloading variations images template
- If user is authenticated then response will be Status Code 200 => OK
Content of the response will be Base-64 encoded array of bytes, that represent the file content. Client needs to decode and use the bytes array.
Name of the file can be found in the response content header Content-Disposition.
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Demo in c# for downloading variations images template
static async Task DownloadingShadowsTemplate() { string token = "test_token"; string url = $"localhost:8080/api/Catalog/Imports/Variations/Images/Template?fileFormat=2";
using (HttpClient client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization =
new AuthenticationHeaderValue(“Bearer”, token);
HttpResponseMessage responseMessage = await client.GetAsync(url);
var content = responseMessage.Content
.ReadAsStringAsync()
.Result;
var contentInBytes = Convert.FromBase64String(content);
System.IO.File.WriteAllBytes($@”C:DataTestRuns{responseMessage.Content.Headers.ContentDisposition.FileName}”, contentInBytes);
}
}
Request for uploading variations images
Endpoint for uploading variations images is:
https://{server_id}.api.sellercloud.com/api/Catalog/Imports/Variations/Images
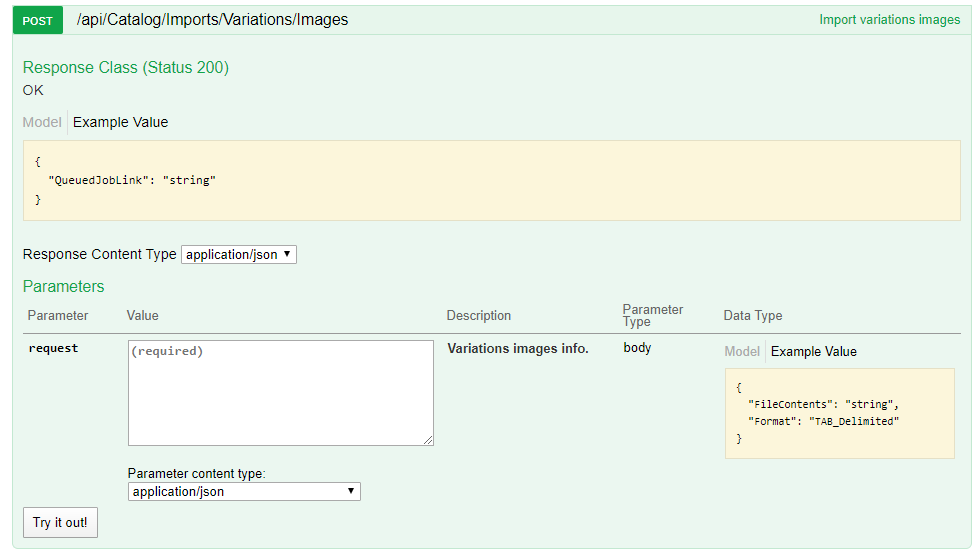
Data | Type | Description | Is Required |
FileContents | string | Base 64 encoded array of bytes | Yes |
Format | int | Format of the file: tab delimited, csv or excel | Yes |