Overview
Endpoint is used for getting product images.
In order to do that, you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
As soon as you do authentication and receive a valid token, it needs to be send in the next call to the API.
Endpoint
NOTE: Endpoint “GET /api/ProductImage/{productID}” is obsolete as it does not support special characters in the productID. Use the new endpoint presented in this article.
Example for such endpoint for TT server is
https://tt.api.sellercloud.com/rest/api/ProductImage
For your server endpoint will be:
https://{your_server_id}.api.sellercloud.com/rest/api/ProductImage
Request
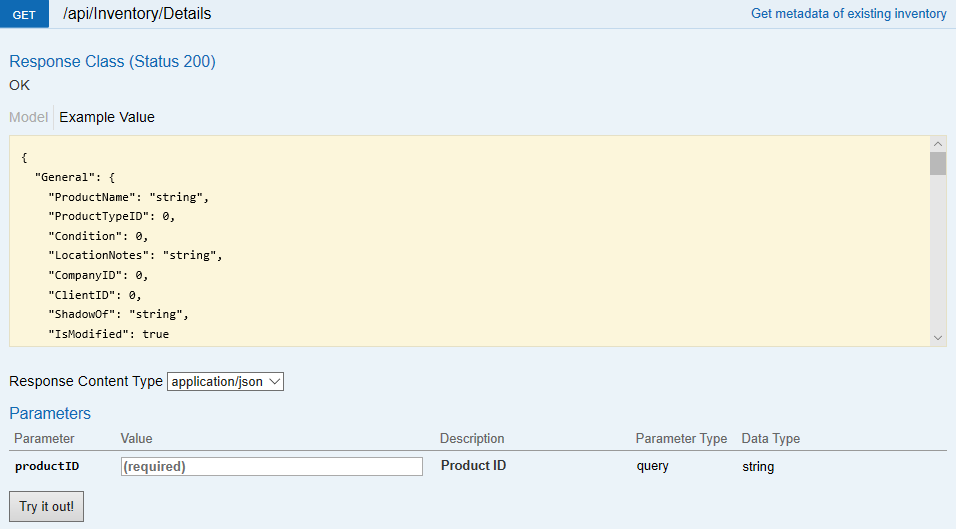
- Method Type: HttpGet
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
- Parameters:
Parameter Data Type Description Is Required productID string ID of existing product. Yes
Response
- If server error appears, then response will be with status code 500 => Internal Server Error
- If product images are retrieved successfully, response code 200 => OK, with list of images data will be provided.
Demo in C#
string token = "test_token"; string url = $"http://cwa.api.sellercloud.com/api/ProductImage?productID=productID"; using (var client = new HttpClient()) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); var responseMessage = client.GetAsync(url).Result; var content = responseMessage.Content .ReadAsStringAsync() .Result; return JsonConvert.DeserializeObject<IList>(content); }
public class ImageDto { public int ImageID { get; set; } public string Url { get; set; } public bool IsDefault { get; set; } public bool IsMainDescriptionImage { get; set; } public string OriginalImageName { get; set; } }