Overview
Endpoint is used for adding product image.
In order to do that, you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
As soon as you do authentication and receive a valid token, it needs to be send in the next call to the API.
Endpoint
Example for such endpoint for TT server is
https://tt.api.sellercloud.com/rest/api/ProductImage
For your server endpoint will be:
https://{your_server_id}.api.sellercloud.com/rest/api/ProductImage
Request
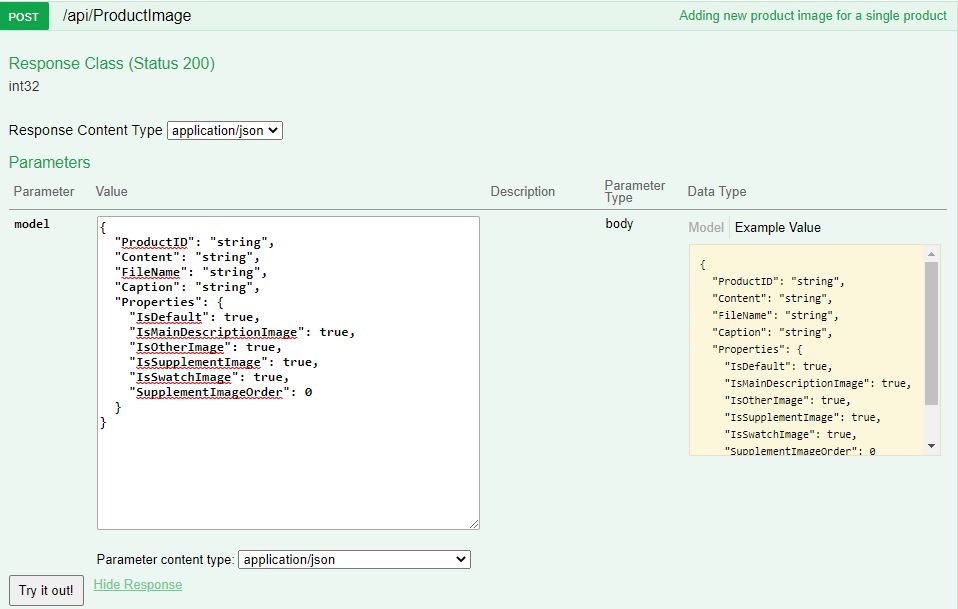
- Method Type: HttpPost
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
- Body data:
Parameter Data Type Description Is Required ProductID string ID of existing product. Yes Content string Base 64 encoded array of bytes. Yes FileName string Name of the file. No Caption string Caption. No Properties object Contains various properties regarding the image No Image Properties
– “IsDefault”: Applys this image as the default product image (Note: if this is the first image you upload for a product, it will be set to default regardless of if the property is true or false)
– “IsMainDescriptionImage”: On certain channels, such as Magento, the description image is assigned to show full size on the product details of a webpage. However, when publishing items to eBay, this full-size image is placed in the HTML product description template.
– “IsSupplementImage”: Images that are neither gallery images nor description images fall under the category of supplemental images. They can be displayed in the product’s extended image gallery. You can have up to 10.
– “IsSwatchImage”:Swatch Images are used by channels to display variations of different colors, fabrics, and other types of product dimensions. Normally, channels that work with Swatch Images accept 1 swatch image per product’s variation.
Request Example
{ "ProductID": "string", "Content": "string", "FileName": "string", "Caption": "string", "Properties": { "IsDefault": true, "IsMainDescriptionImage": true, "IsOtherImage": true, "IsSupplementImage": true, "IsSwatchImage": true, "SupplementImageOrder": 0 } }
Response
- If server error appears, then response will be with status code 500 => Internal Server Error
- If image is added successful, response will be status code 200 => Ok and response will be ID of the newly added image.
Demo in C#
string token = "test_token"; string url = $"http://cwa.api.sellercloud.com/api/ProductImage"; string fileName = $@"C:FilePathimage.jpg"; var content = new AddProductImageRequest() { ProductID = "0003A", Content = Convert.ToBase64String(File.ReadAllBytes(fileName)), FileName = fileName, Properties = new ProductImageProperties { IsDefault = true, IsMainDescriptionImage= true, IsOtherImage= false, IsSupplementImage= true, IsSwatchImage= true, SupplementImageOrder= 2, } }; using (var client = new HttpClient()) using (var request = new HttpRequestMessage(HttpMethod.Post, url)) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); var json = JsonConvert.SerializeObject(content); using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json")) { request.Content = stringContent; await client.SendAsync(request); } }