Overview
Endpoint is used for updating value of user defined PO column. In order to do that you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
Endpoint
Example for such endpoint for TT server is https://tt.api.sellercloud.com/api/PurchaseOrders/{id}/CustomColumns
For your server endpoint will be:
https://{your_server_id}.api.sellercloud.com/api/PurchaseOrders/{id}/CustomColumns
Request
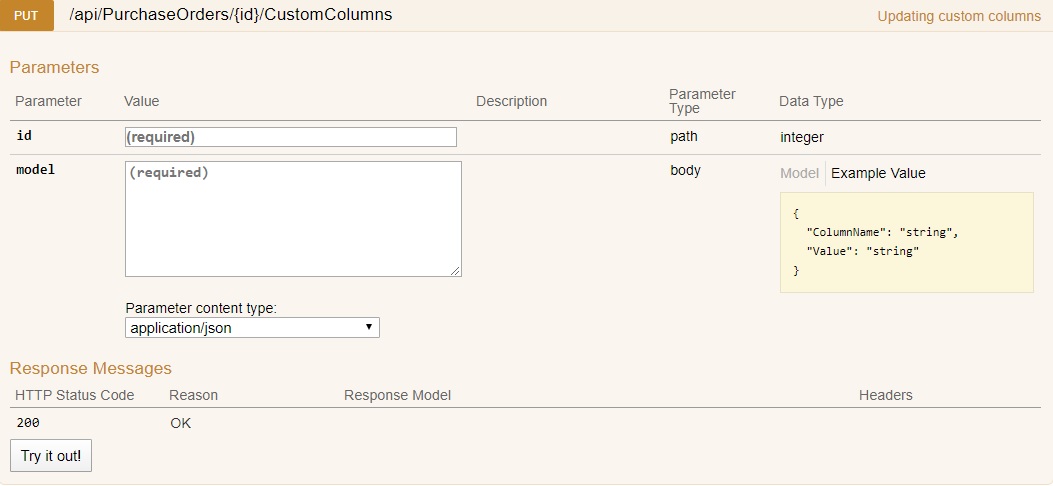
- Method Type: HttpPut
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
- Body data:
Parameter Data Type Description Is Required ColumnName string Name of existing column name. Mandatory. Yes Value string Value of custom columns. Mandatory. Yes
Example Request Payload
{ "ColumnName": "Some_Custom_Column", "Value": "Test value" }
Response
- If server error appears, then response will be with status code 500 => Internal Server Error
- If updating value of custom column is successful, status code will be OK 200
Demo in c#
string token = "test token"; string url = $"http://cwa.api.sellercloud.com/api/PurchaseOrders/1/CustomColumns"; var content = new UpdateCustomColumnRequest() { ColumnName = "TEST", Value = "TEST VALUE" }; using (var client = new HttpClient()) using (var request = new HttpRequestMessage(HttpMethod.Put, url)) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); var json = JsonConvert.SerializeObject(content); using (var stringContent = new StringContent(json, Encoding.UTF8, "application/json")) { request.Content = stringContent; HttpResponseMessage responseMessage = await client.SendAsync(request); } }