Overview
Download a template for importing Walmart Marketplace product Attributes and update your products. Note that the template and available attributes for Walmart Marketplace vary based on Country.
In order to call these endpoints, you must:
- Be an authenticated user
For information on how you can authenticate, see Authentication
As soon as you do authentication and receive a valid token, it needs to be passed on the calls to the server.
Endpoints
An example of Download Template endpoint for XX server is
https://XX.api.sellercloud.com/rest/api/Catalog/Imports/WalmartMarketplaceAttributes/Template
An example of Update Attributes endpoint for XX server is
https://XX.api.sellercloud.com/rest/api/Catalog/Imports/WalmartMarketplaceAttributes
Request for Downloading Attributes Template
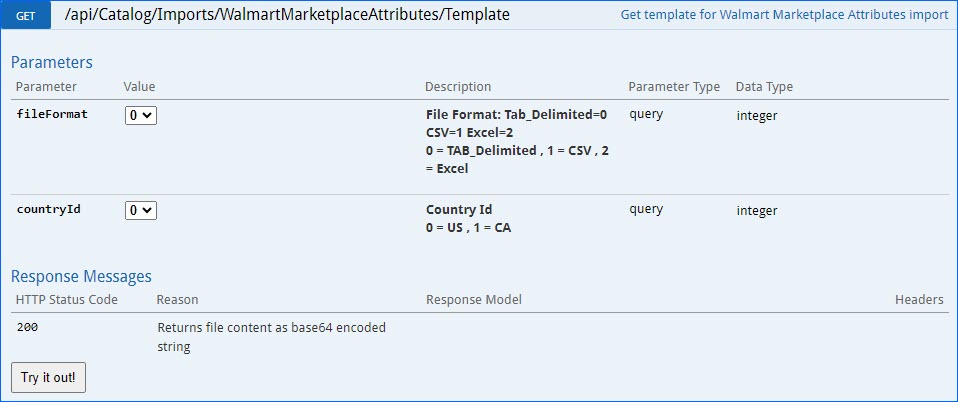
enum FileFormatType { TAB_Delimited = 0 CSV = 1 Excel = 2 }
Response for Downloading Attributes Template
- If the user is authenticated, then the response will be Status Code 200 => OK
The content of the response will be a Base-64 encoded array of bytes that represent the file content. You need to decode and use the bytes array.
The name of the file can be found in the response content header Content-Disposition.
- If the user is not authenticated, then the response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
- In case of an incorrect request, the response will be with Status Code 400 Bad Request
Demo in c# for Downloading Attributes Template
static async Task DownloadingWalmartMarketplaceAttributesTemplate() { string token = "test_token"; string url = $"localhost:8080/api/Catalog/Imports/WalmartMarketplaceAttributes?fileFormat=2"; using (HttpClient client = new HttpClient()) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); HttpResponseMessage responseMessage = await client.GetAsync(url); var content = responseMessage.Content .ReadAsStringAsync().Result; var contentInBytes = Convert.FromBase64String(content); System.IO.File.WriteAllBytes($@"C:\DataTestRuns{responseMessage.Content.Headers.ContentDisposition.FileName}", contentInBytes); } }
Request for Importing Attributes
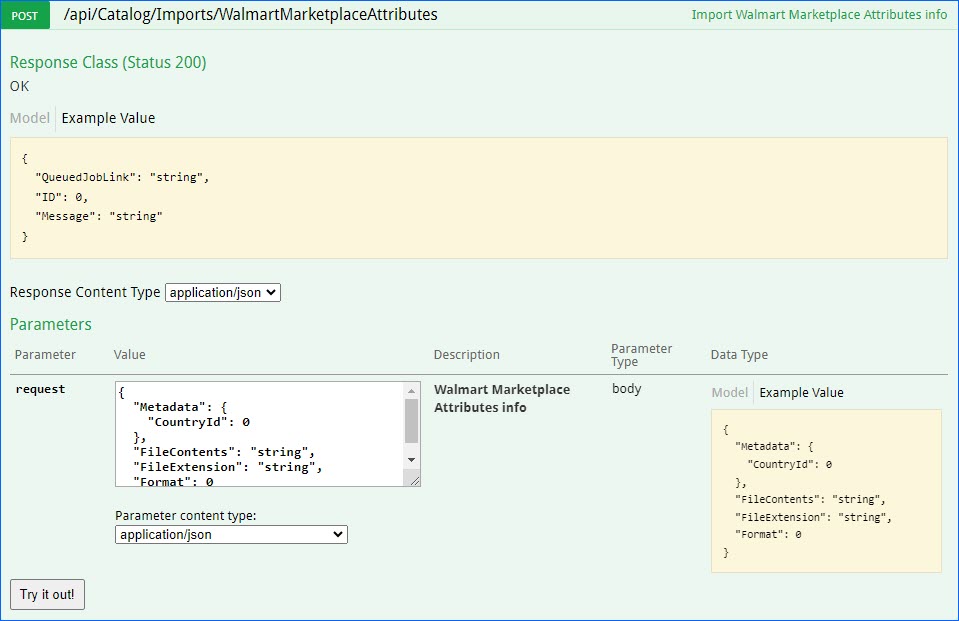
Parameter | Data Type | Description | Is Required |
CountryId | enum | The country of your listings’ Walmart Account: 0 = US , 1 = CA |
Yes |
FileContents | string | Base 64 encoded array of bytes. | Yes |
FileExtension | string | The imported file’s extension. If the file format is Excel, you must provide file extension: xls or xlsx | No |
Format | enum | Format of the file: Tab_Delimited=0 CSV=1 Excel=2 | Yes |
Request Format
{ "Metadata": { "CountryId": 0 }, "FileContents": "string", "FileExtension": "string", "Format": 0 }
Response for Importing Attributes
- If the user is authenticated and the request is successful, then the response will be Status Code 200 => OK
{ "QueuedJobLink": "string", "ID": 0, "Message": "string" }
- If the user is not authenticated, then the response will be Status Code 401 => Not Valid Token
- In case of an error, the response will be Status Code 500 => Internal Server Error
- In case of an incorrect request, the response will be with Status Code 400 Bad Request