Overview
In order to get information for execution history of a scheduled task, you can consume the endpoint presented in this article. In order to consume it, you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
You must also know the ID of a schedule task. This can be seen from manage scheduled tasks page in Delta UI.
Endpoint
Example for such endpoint for TT server is:
https://tt.api.sellercloud.com/rest/api/ScheduledTasks/{id}/ExecutionHistory
For your server endpoint will be:
https://{your_server_id}.api.sellercloud.com/rest/api/ScheduledTasks/{id}/ExecutionHistory
Request
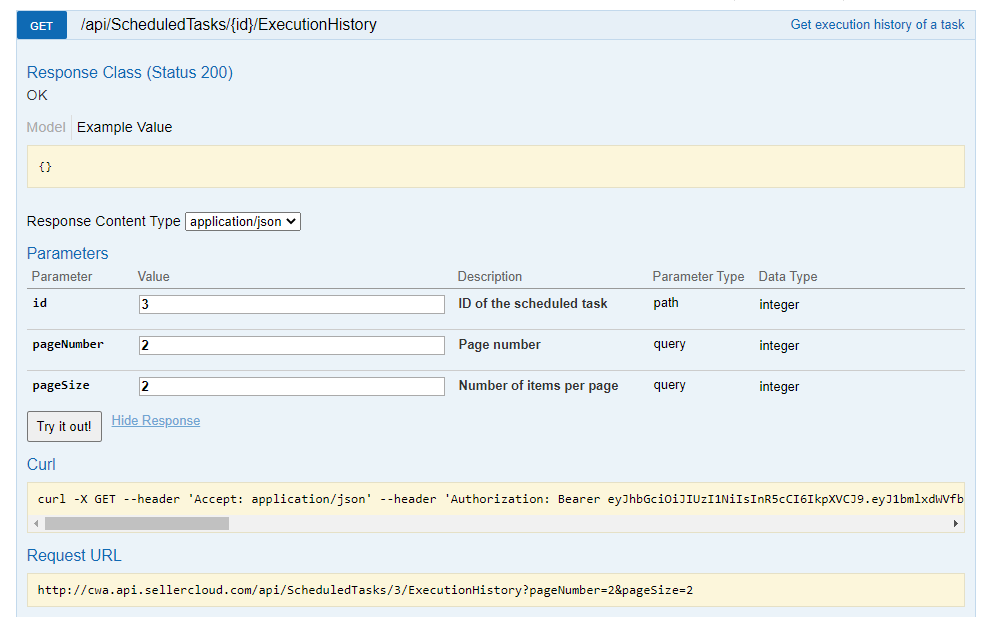
- Method Type: HttpGet
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
- Parameters: ID of the existing schedule task
Parameter | Data Type | Description | Is Required |
id | integer | ID of existing task | Yes |
pageNumber | integer | Page number | Yes |
pageSize | integer | Page size | Yes |
Example:
https://tt.api.sellercloud.com/rest/api/ScheduledTasks/1/ExecutionHistory?pageNumber=1&pageSize=10
Response
- If user is authenticated and provides a valid ID of a scheduled task, then response will be Status Code 200 => OK and history metadata in JSON format
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Example Response
{ "Results": [ { "ExecutedOn": "", "JobID": 32, "ExecutedBy": "System", "Status": "Submitted" "StartedOn": null, "CompletedOn": null, "Error": "", "Duration": "", "UserID": 0 } ], "TotalResults": 1175 }
Demo in C#
static async Task Main(string[] args) { await GetExecutionHistory(); } static async Task GetExecutionHistory() { // Valid ID of a scheduled task can be found through Delta UI int task = 28142; // URL for downloading execution history string url = $"http://localhost:64158/api/ScheduledTasks/{jobID}/ExecutionHistory?pageNumber=1&pageSize=10"; // Valid token must be provided here string token = "test token"; using (HttpClient client = new HttpClient()) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); HttpResponseMessage responseMessage = await client.GetAsync(url); var content = responseMessage.Content; } }
public class ExecutionHistoryItem { public DateTime? ExecutedOn { get; set; } public int JobID { get; set; } public string ExecutedBy { get; set; } public string Status { get; set; } public DateTime? StartedOn { get; set; } public DateTime? CompletedOn { get; set; } public string Error { get; set; } public string Duration { get; set; } public string UserID { get; set; } }
public class ExecutionHistory { public IEnumerable Results { get; set; } public int TotalResults { get; set; } }