Overview
In order to get information for output file of a single queued job, you can consume the endpoint presented in this article. In order to consume it, you must:
- Be authenticated user
For information on how you can authenticate, see: Authentication
As soon as you do authentication and receive a valid token, it needs to be passed on the call for receiving a single queued job information.
- Have valid ID of an existing queued job
Endpoint
Example for such endpoint for TT server is:
https://tt.api.sellercloud.com/rest/api/QueuedJobs/OutputFile/{id}
For your server endpoint will be:
https://{your_server_id}.api.sellercloud.com/rest/api/QueuedJobs/OutputFile/{id}
Request
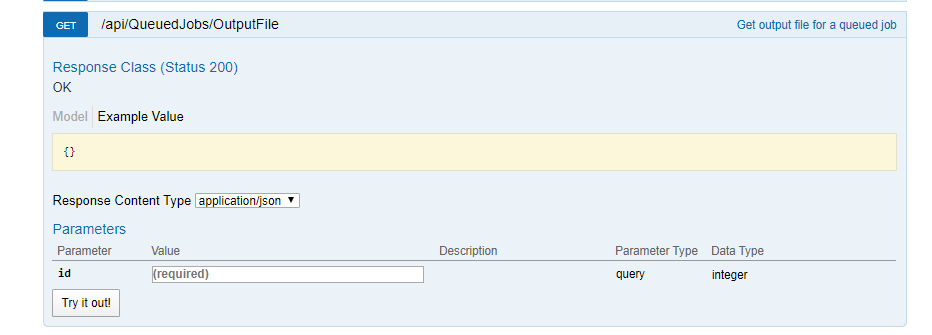
- Method Type: HttpGet
- Authorization: Use Bearer Token + token received from token authentication
- Header info: Content-Type: application/json
- Parameters: ID of the existing queued job
Parameter | Data Type | Description | Is Required |
id | integer | ID of existing queued job | Yes |
Example: https://tt.api.sellercloud.com/rest/api/queuedjobs/outputfile/1
Response
- If user is authenticated and provides a valid ID of job, then response will be Status Code 200 => OK and encoded array of bytes, which represent the file content.That response content should be decoded from base-64 and array of bytes can be used to save and open the file.
Name of the file and its extension are stored in the content header as ContentDisposition item
- If user is not authenticated, then response will be Status Code 401 => Not Valid Token
- On server response => Status Code 500 => Internal Server Error
Demo in c#
static async Task Main(string[] args) { await GetOutputFile(); Console.WriteLine("Output file successfully downloaded!"); } static async Task GetOutputFile() { // Valid ID of a queued job must be provided here int jobID = 28142; // URL for downloading output file of a queued job string url = $"http://localhost:64158/api/QueuedJobs/OutputFile?id={jobID}"; // Valid token must be provided here string token = "test token"; using (HttpClient client = new HttpClient()) { client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", token); HttpResponseMessage responseMessage = await client.GetAsync(url); var content = responseMessage.Content .ReadAsStringAsync() .Result; var contentInBytes = Convert.FromBase64String(content); System.IO.File.WriteAllBytes($@"C:DataTestRuns{responseMessage.Content.Headers.ContentDisposition.FileName}", contentInBytes); } }